mirror of
https://github.com/Aust1n46/VentureChat.git
synced 2025-05-23 02:19:05 +00:00
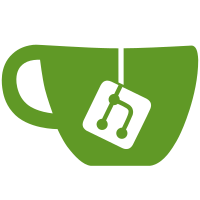
Implement DI framework and reduce static abuse Improve testability Remove deprecated features
63 lines
1.4 KiB
Java
63 lines
1.4 KiB
Java
package venture.Aust1n46.chat;
|
|
|
|
import org.slf4j.LoggerFactory;
|
|
|
|
import com.google.inject.Inject;
|
|
|
|
public class Logger {
|
|
private static final String LOG_PREFIX = "[VentureChat] ";
|
|
|
|
private org.slf4j.Logger parent = LoggerFactory.getLogger(Logger.class);
|
|
|
|
@Inject
|
|
private VentureChat plugin;
|
|
|
|
public void info(String message) {
|
|
parent.info(LOG_PREFIX + message);
|
|
}
|
|
|
|
public void info(String format, Object arg) {
|
|
parent.info(LOG_PREFIX + format, arg);
|
|
}
|
|
|
|
public void info(String format, Object arg1, Object arg2) {
|
|
parent.info(LOG_PREFIX + format, arg1, arg2);
|
|
}
|
|
|
|
public void info(String format, Object... arguments) {
|
|
parent.info(LOG_PREFIX + format, arguments);
|
|
}
|
|
|
|
public void debug(String message) {
|
|
if (plugin.getConfig().getString("loglevel", "info").equals("debug")) {
|
|
info(message);
|
|
}
|
|
}
|
|
|
|
public void debug(String format, Object arg) {
|
|
if (plugin.getConfig().getString("loglevel", "info").equals("debug")) {
|
|
info(format, arg);
|
|
}
|
|
}
|
|
|
|
public void debug(String format, Object arg1, Object arg2) {
|
|
if (plugin.getConfig().getString("loglevel", "info").equals("debug")) {
|
|
info(format, arg1, arg2);
|
|
}
|
|
}
|
|
|
|
public void debug(String format, Object... arguments) {
|
|
if (plugin.getConfig().getString("loglevel", "info").equals("debug")) {
|
|
info(format, arguments);
|
|
}
|
|
}
|
|
|
|
public void warn(String message) {
|
|
parent.warn(message);
|
|
}
|
|
|
|
public void error(String message) {
|
|
parent.error(message);
|
|
}
|
|
}
|